
Header and footer sections in a document show document information, such as the title of the document, logo, the chapter heading, page numbers, etc. We can add any text or image in the headers/footers of the PDF document programmatically. In this article, we will learn how to add headers and footers in PDF documents using C#.
The following topics shall be covered in this article:
- C# API to Add Headers and Footers in PDF Documents
- Add Text in Header of PDF using C#
- Add Text in Footer of PDF using C#
- Insert Image in Header of PDF using C#
- Insert Image in Footer of PDF using C#
- Add Different Headers and Footers on Different Pages
- Add Page Numbers in Footer of PDF using C#
C# API to Add Headers and Footers in PDF Documents
For adding headers and footers in PDF files, we will be using Aspose.PDF for .NET API. It allows us to generate, modify, convert, render, secure and print supported documents without using Adobe Acrobat. Please either download the DLL of the API or install it using NuGet.
Install-Package Aspose.PDF
Add Text in Header of PDF using C#
We can add text in the header of an existing PDF document by following the steps given below:
- Firstly, load a PDF document using the Document class with input file path as an argument. It is the main class that represents a PDF document and allows performing various functionalities.
- Next, create an instance of the TextStamp class with a text to show in the header of the document.
- Then, set various properties such as TopMargin, HorizontalAlignment, and VerticalAlignment as Top, etc.
- Optionally, set ForegroundColor, Font, FontStyle, FontSize, BackgroundColor, RotateAngle and Zoom level for the text.
- After that, loop through all the pages and add header using the Page.AddStamp() method with TextStamp object.
- Finally, call the Document.Save() method with the output file path as an argument to save the output file.
The following code sample shows how to add text in the header of a PDF document using C#.
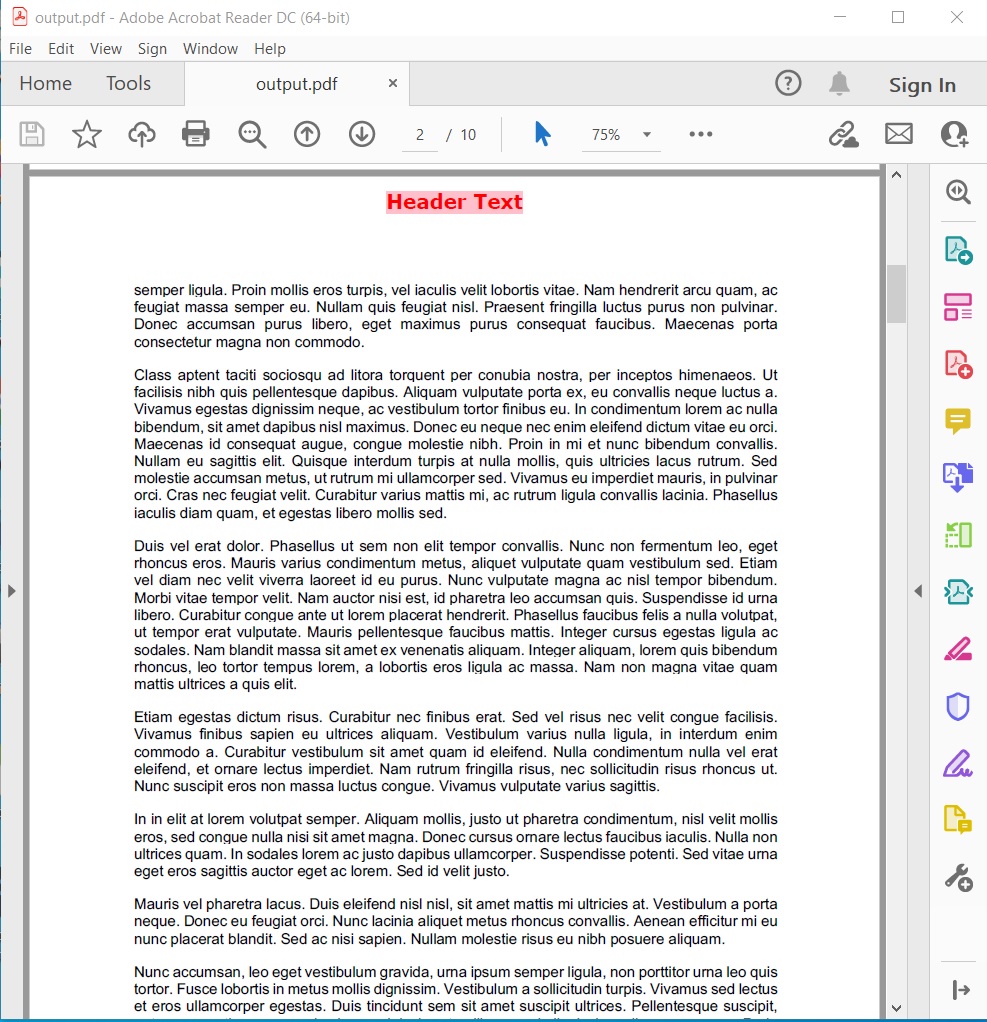
Add Text in Header of PDF using C#.
Add Text in Footer of PDF using C#
We can add text in the footer of PDF documents programmatically by following the steps mentioned earlier. However, we need to set BottomMargin and VerticalAlignment as Bottom to show the text in the footer.
The following code sample shows how to add text in the footer of a PDF document using C#.
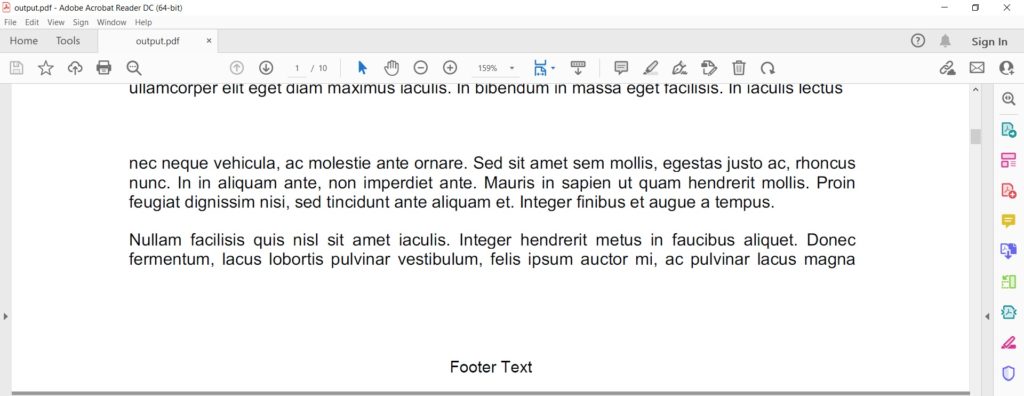
Add Text in Footer of PDF using C#.
Insert Image in Header of PDF using C#
We can also add an image in the header of an existing PDF document by following the steps given below:
- Firstly, load a PDF document using the Document class with input file path as an argument.
- Next, create an instance of the ImageStamp class with image file path as an argument.
- Then, set various properties such as TopMargin, HorizontalAlignment, and VerticalAlignment as Top, etc.
- After that, loop through all the pages and add header using the Page.AddStamp() method with ImageStamp object.
- Finally, call the Document.Save() method with the output file path as an argument to save the output file.
The following code sample shows how to add an image in the header of a PDF document using C#.

Insert Image in Header of PDF using C#.
Insert Image in Footer of PDF using C#
We can add images in the footer of PDF documents programmatically by following the steps mentioned earlier. However, we need to set BottomMargin and VerticalAlignment as Bottom to show the image in the footer.
The following code sample shows how to add an image in the footer of a PDF document using C#.
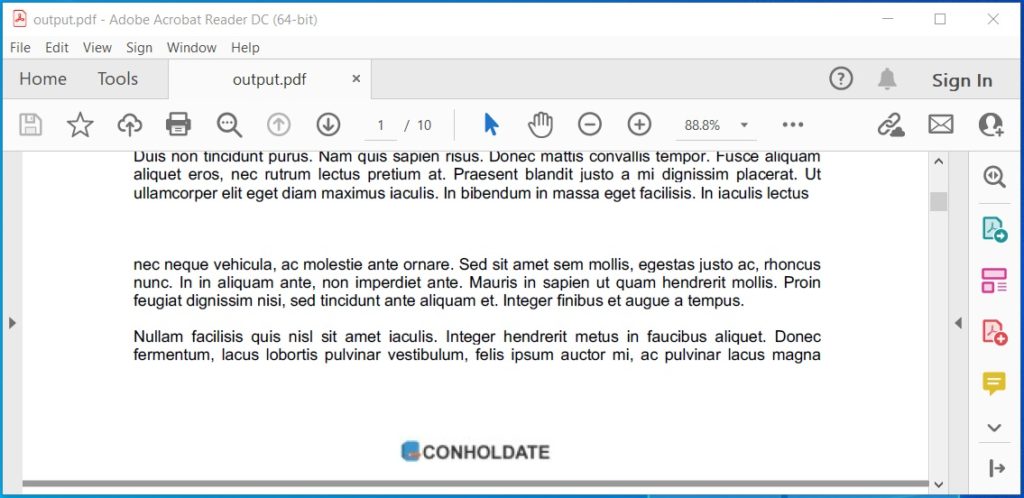
Insert Image in Footer of PDF using C#.
Add Different Headers and Footers on Different Pages
We can add different headers/footers for different pages in a single PDF document by following the steps given below:
- Firstly, load a PDF document using the Document class with input file path as an argument.
- Next, create multiple instances of the ImageStamp class with image file path and/or the TextStamp class with a text to show.
- Then, set various properties such as TopMargin, HorizontalAlignment, and VerticalAlignment as Top for the header and BottomMargin and VerticalAlignment as Bottom for the footer.
- After that, add header or footer using the Page.AddStamp() method with ImageStamp or TextStamp object for a page.
- Finally, call the Document.Save() method with the output file path as an argument to save the output file.
The following code sample shows how to add multiple headers and footers in a single PDF document using C#.
Add Page Numbers in Footer of PDF using C#
We can add page numbers in the footer section of PDF documents by following the steps given below:
- Firstly, load a PDF document using the Document class with input file path as an argument.
- Next, do the following for each page in the Document.Pages collection.
- Create an instance of the TextStamp class with a text concatenated with the current page number.
- Then, set various properties such as BottomMargin, HorizontalAlignment, and VerticalAlignment as Bottom, etc.
- After that, call the Page.AddStamp() method with the TextStamp object to add page number in the footer.
- Finally, call the Document.Save() method with the output file path as an argument to save the output file.
The following code sample shows how to add a page number for each page in the footer of a PDF document using C#.
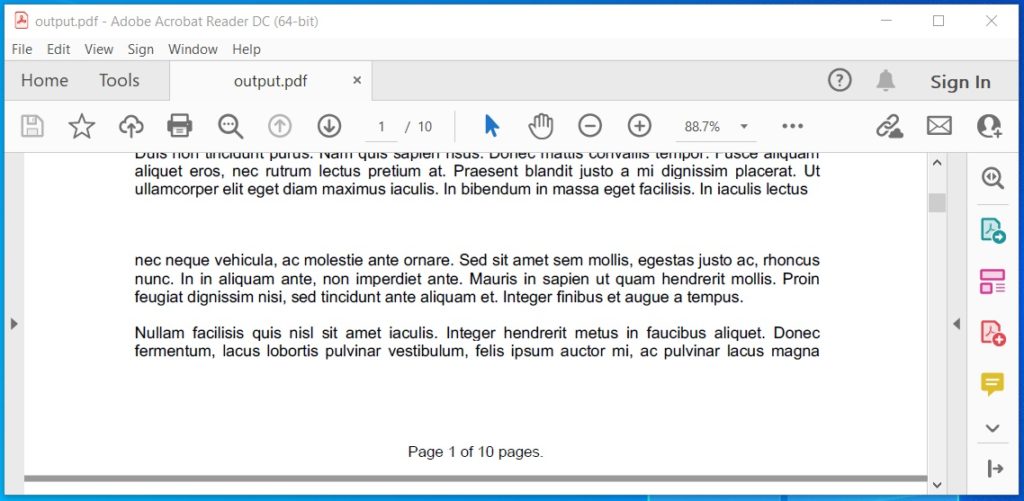
Add Page Numbers in Footer of PDF using C#.
Get a Free API License
You can try the API without evaluation limitations by requesting a free temporary license.
Conclusion
In this article, we have learned how to add a text or an image in the headers/footers of existing PDF files using C#. We have also seen how to add different headers on different pages in a PDF document and how to add a page number in the footer of a document. Besides, you can learn more about Aspose.PDF for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.