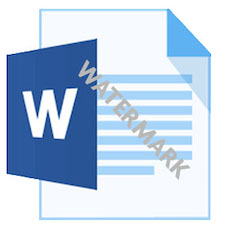
As a C# developer, you can easily add text or image watermarks in Word documents programmatically. A watermark is a sort of message in the form of text or an image, usually used to identify or protect the documents by showing copyright information, disclaimer, logo, stamp, or signature. In this article, you will learn how to add text or image watermarks in Word documents using C#.
The following topics are discussed/covered in this article:
- C# API to Add Watermark in Word Documents
- Add Text Watermark in Word Documents using C#
- Add Image Watermark in Word Documents using C#
- Watermark the Images of a Word Document using C#
- Add Watermark to Specific Pages in Word Documents using C#
- Add Watermark to Header or Footer of Word Documents using C#
C# API to Add Watermark in Word Documents
For adding text or image watermarks in DOC or DOCX files, we will be using GroupDocs.Watermark for .NET API. It enables you to add, edit, search and remove image and text watermarks in supported file formats. It also allows obtaining the basic information about source documents such as file type, size, pages count, page height and width, etc. The document preview feature of the API allows generating image representations of document pages for a better understanding of the document.
You can either download the DLL of the API or install it using NuGet.
Install-Package GroupDocs.Watermark
Add Text Watermark in Word Documents using C#
You can add a text watermark to Word documents by following the steps given below:
- Firstly, load the DOCX file using the Watermarker class.
- Initialize the font to be used for watermark text using the Font class.
- Create an instance of the TextWatermark class to create a text watermark. Pass the text to show as watermark and defined font object as input parameters.
- Now, set various watermark properties such as Foreground Color, Background Color, Rotate Angle, Height, Width, Opacity, etc.
- Then, call the Watermarker.Add() method to add the text watermark to the document.
- Finally, call the Watermarker.Save() method to save the watermarked Word document.
The following code sample shows how to add a text watermark in a DOCX file using C#.
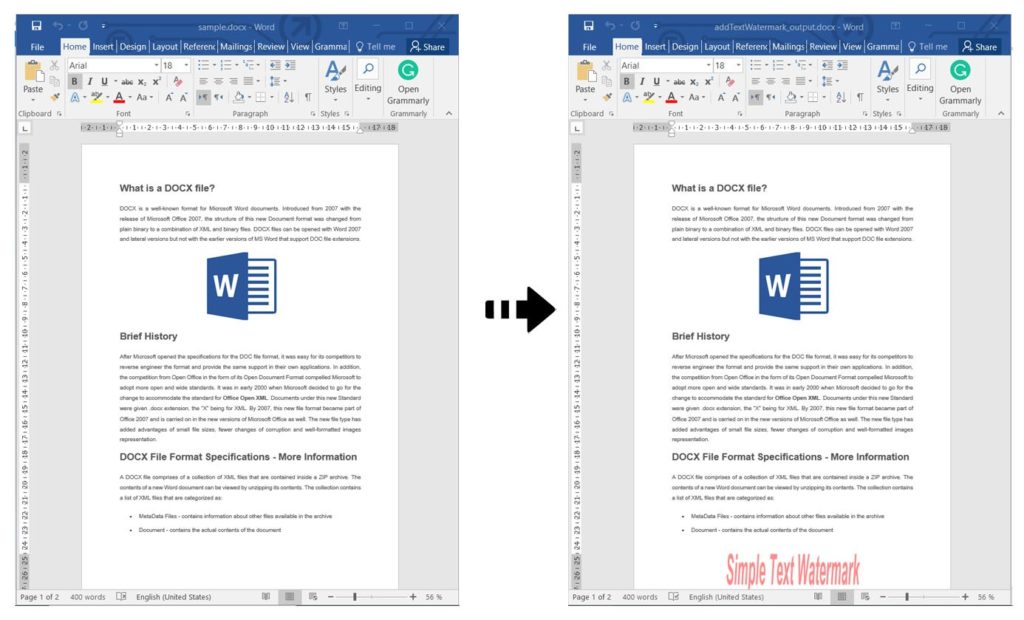
Add Text Watermark in Word Documents using C#
Add Image Watermark in Word Documents using C#
You can add an image as a watermark to Word documents by following the steps given below:
- Firstly, load the DOCX file using the Watermarker class.
- Create an instance of the ImageWatermark class with the image path to create an image watermark.
- Now, set various watermark properties such as Alignment, Height, Width, etc.
- Then, call the Watermarker.Add() method to add the image watermark to the document.
- Finally, call the Watermarker.Save() method to save the watermarked Word document.
The following code sample shows how to add an image watermark in a DOCX file using C#.
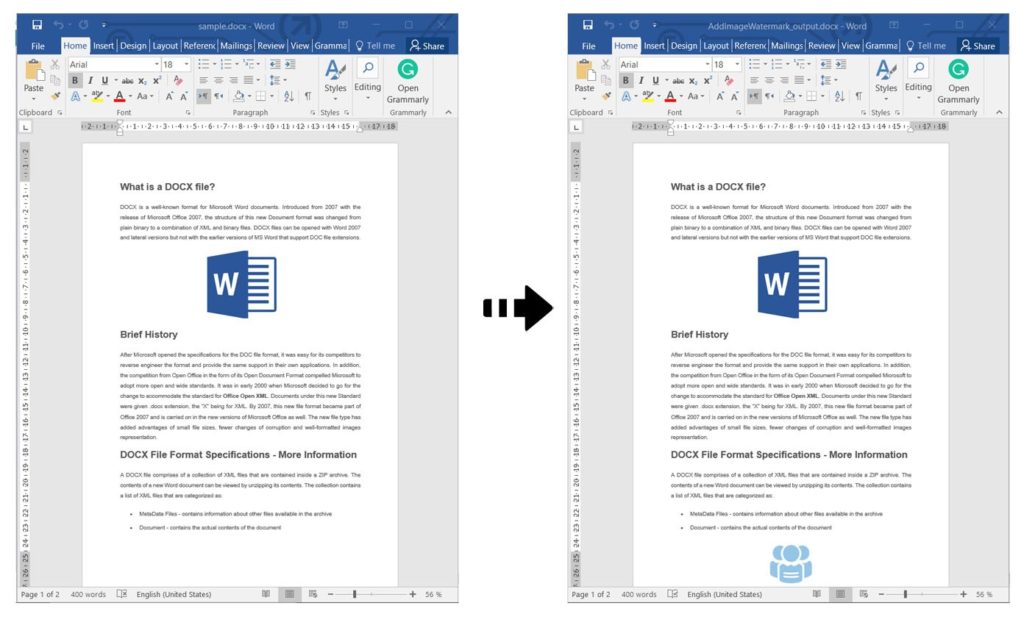
Add Image Watermark in Word Documents using C#
Watermark the Images of a Word Document using C#
You can add text watermark to the images in Word documents by following the steps given below:
- Firstly, load the DOCX file using the Watermarker class.
- Create an instance of the TextWatermark class to create a text watermark. Pass the text to show as watermark and the font to be used for watermark text using the Font class as input parameters.
- Now, set various watermark properties e.g. Foreground Color, Alignment, Rotate Angle, Scale Factor, etc.
- Then, Call the Watermarker.GetImages() method to find all images in the document and get results in the WatermarkableImageCollection class object.
- For each image in WatermarkableImageCollection, add watermark by calling the WatermarkableImage.Add() method with the TextWatermark object.
- Finally, call the Watermarker.Save() method to save the watermarked Word document.
The following code sample shows how to add a text watermark to the images in a DOCX file using C#.
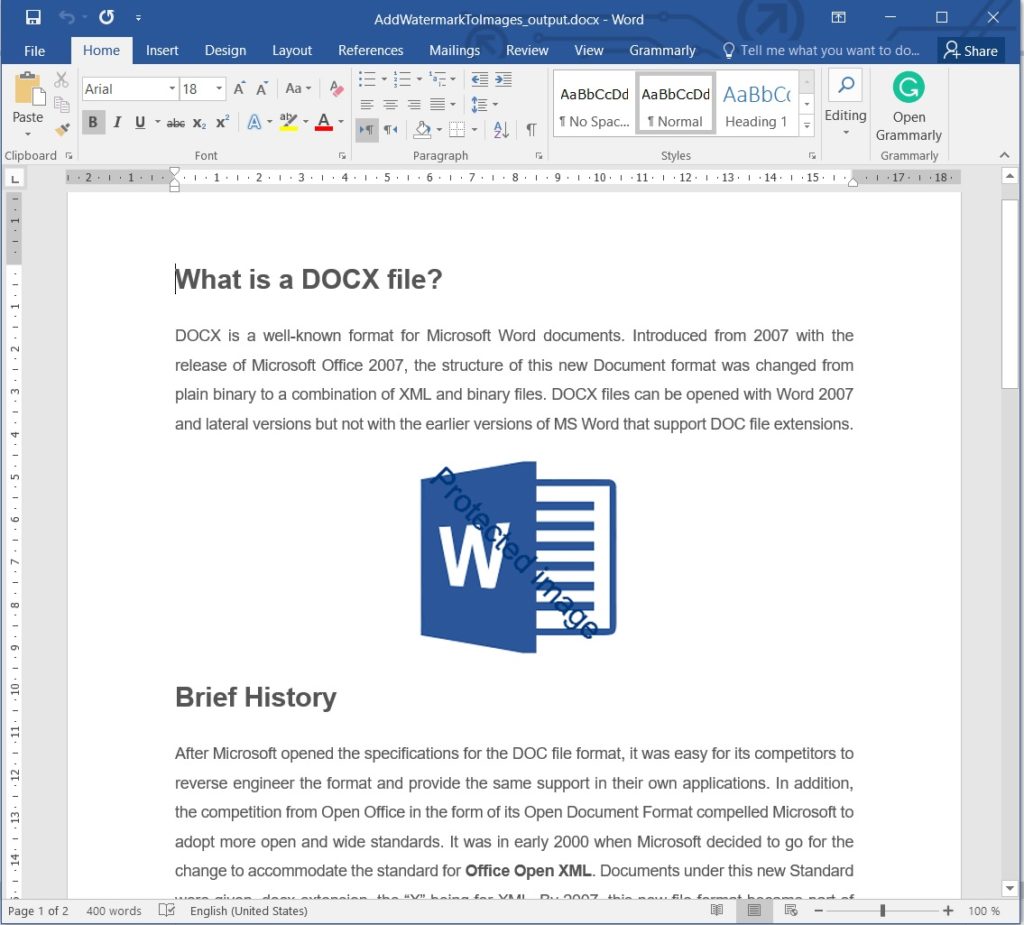
Watermark Images in Word Documents using C#.
Add Watermark to Specific Pages in Word Documents using C#
You can add watermark to a specific page of the Word document by following the steps given below:
- Firstly, load the DOCX file using the Watermarker class.
- Initialize the font to be used for watermark text using the Font class.
- Create an instance of the TextWatermark class to create a text watermark. Pass the text to show as watermark and defined font object as input parameters.
- Now, set various watermark properties such as Foreground Color, Background Color, Alignment, etc.
- Create an instance of the WordProcessingWatermarkPagesOptions class
- Now, set the PageNumbers to add the watermark. You can set a single page number or a comma separated list of page numbers. We set it to the WordProcessingContent.PageCount which indicates the last page of the document here.
- Then, call the Watermarker.Add() method to add the defined watermark.
- Finally, call the Watermarker.Save() method to save the watermarked Word document.
The following code sample shows how to add a text watermark to a specific page in a DOCX file using C#.
Add Watermark to Header or Footer of Word Documents using C#
You can add a watermark to the header or footer sections of the Word documents by following the steps given below:
- Firstly, load the DOCX file using the Watermarker class.
- Create an instance of the ImageWatermark class with the image path to create an image watermark.
- Then, set various watermark properties such as Alignment, Height, Width, etc.
- Create an instance of the WordProcessingWatermarkSectionOptions class.
- Now, set the WordProcessingWatermarkSectionOptions.SectionIndex to 0 to add watermark to the first section of the document.
- Then, call the Watermarker.Add() method to add the image watermark to first section.
- Call the Watermarker.GetContent() method to get the content for the loaded document and get results in the WordProcessingContent class object.
- Loop through all the sections and call the LinkToPrevious() method with true boolean value as input parameter. It will link all headers and footers of all the sections with the first section.
- Finally, call the Watermarker.Save() method to save the watermarked Word document.
The following code sample shows how to add a watermark to a header or footer section in a DOCX file using C#.
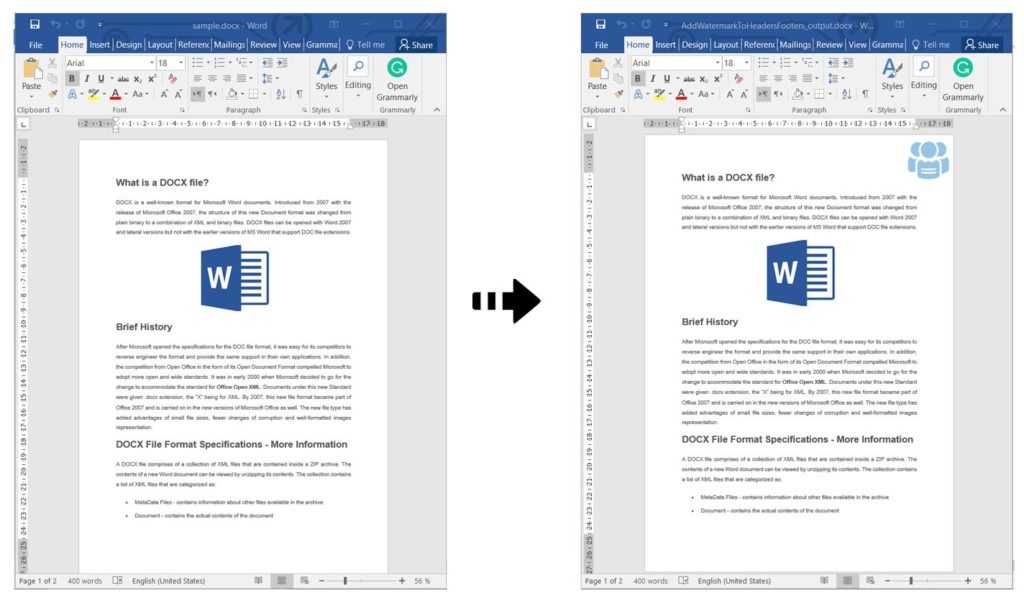
Add Watermark to Header or Footer of Word Documents using C#.
Get a Free License
You can try the API without evaluation limitations by requesting a free temporary license.
Conclusion
In this article, you have learned how to add text or image watermark in the Word documents using C#. Moreover, you have seen how to add watermark to a specific page of a Word document programmatically. This article also explained how to add watermark to images in the DOCX file using C#. Besides, you can learn more about GroupDocs.Watermark for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.