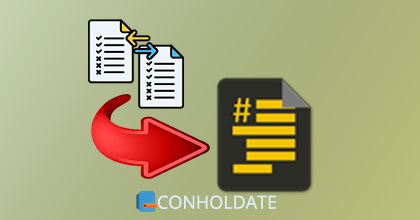
Compare and Merge Source Code Files in Java
As a Java developer, if you are going to build a source code comparison tool or application or you may need to manage several versions of code files then you may think about how to compare and merge source code files in Java. If so, this article will explain how easily we can do this without writing a number of lines of code. Besides, we shall see how we can retrieve, accept or discard the changes while merging the source code files.
This article will cover the points given below:
- Java library to compare and merge source code files
- How to compare and merge source code files in Java
- Get the list of changes using Java
- Advance options in Java code comparison library
Java library to compare and merge source code files
To compare and merge source code files, we shall use Java code comparison library which consists of all important and outstanding source code comparison features. This Java code comparing library can either be downloaded or can be installed directly by using the Maven configuration as given below.
<repository>
<id>groupdocs-artifacts-repository</id>
<name>GroupDocs Artifacts Repository</name>
<url>https://repository.groupdocs.com/repo/</url>
</repository>
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-comparison</artifactId>
<version>22.11</version>
</dependency>
How to compare and merge source code files in Java
Suppose we have two files (source.cs and target.cs) of different versions of a source code as shown in the following screenshot.
By considering the files above, let’s write a code snippet in Java by following the steps as given below:
- Initialize Comparer class with the source document’s path or stream.
- Invoke add() method and specify target document’s path or stream.
- Invoke compare() method.
The following code sample shows how easily you can compare and merge source code files in Java.
As a result, we shall get the merged source code file and an HTML file highlighting the changes in the code. Please note that the deleted elements will be marked in red, the added – in blue, and the modified – in green.
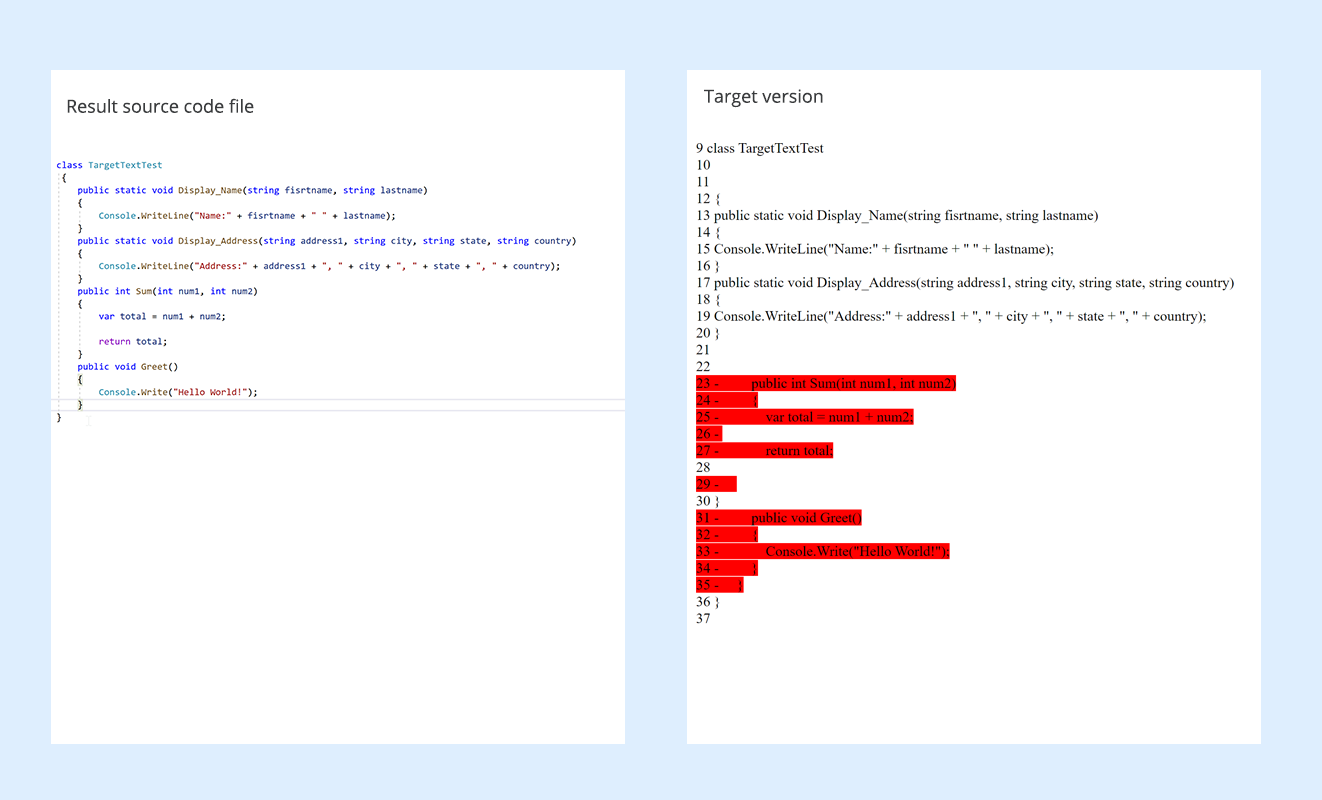
Get the list of changes using Java
To get the list of all changes after comparison, we shall write the code as per the following steps:
- Initialize Comparer object with source file’s path.
- Invoke add() method and specify target file’s path.
- Invoke compare() method.
- Get the list of changes by using getChanges() method.
The code sample below demonstrates how to get the list of all changes.
Advance options in Java code comparison library
If you need to accept or reject several versions of code, you may have to apply or discard the changes. In this case, please follow the steps given below:
- Initiallize object of Comparer class with the source document’s path or stream.
- Call add() method and set target document’s path.
- Invoke compare() method.
- Invoke getChanges() method and obtain detected changes list.
- Set ComparisonAction of needed change object to ComparisonAction.ACCEPT or ComparisonAction.REJECT value.
- Call applyChanges() method and pass collection of changes to it.
The following code snippet shows how to use the advanced options of the code comparison library.
Get a Free License
You can get a free temporary license to try the API without evaluation limitations.
Conclusion
To sum up, we hope that you have learned how to compare and merge source code files programmatically using a Java code comparison library. As a result, you have got the summary of inserted or deleted content. Furthermore, you have gone through the ways to get the changes list and accept or reject the identified changes.
You may visit the documentation to explore other features.
Ask a question
You can let us know your questions or queries on our forum.
FAQs
How to compare and merge source code files in Java?
Initialize Comparer class with source document path or stream, call add() method, and set the target document’s path or stream. After that, invoke compare() method of Comparer class.