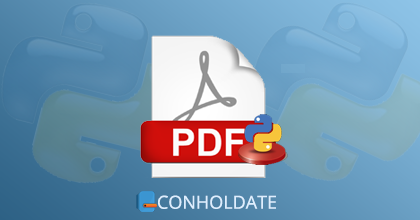
PDF file format is commonly used to present and exchange documents. In addition, it provides many benefits, such as reducing MBs and rendering text, images, tables, annotations, bookmarks, and hyperlinks without losing layout and formatting. Python developers may consider these advantages and look to publish their data as PDFs. If you are one of those developers, this article will help you to learn how to create a PDF document using Python.
The following points will be covered in this article:
- Python PDF Creator Library - Installation
- Generate a PDF Document using Python
- Apply Text Formatting in PDFs
- Insert Images in a PDF file
- Add Tables in PDFs
Installing Python PDF Creator Package
To create a PDF document programmatically, we will use a Python PDF creator package, which allows developers to generate, read, convert, and manipulate PDF files without using Adobe Acrobat.
Please download or install the package from PyPI using the pip command given below:
pip install aspose-pdf
How to Easily Create a PDF Document in Python
Firstly, we are going to explain how to create a PDF document containing a text fragment. The following are the steps to create a simple PDF document from scratch.
- Create an instance of Document class.
- Add a new Page to the pages collection of the document.
- Create and add a new TextFragment to the paragraphs of the PDF.
- Generate the PDF file using Document.Save() method.
The following code snippet demonstrates how to create a PDF document in Python.
You will see the output as follows.
Apply Text Formatting in PDFs using Python
After creating a document let’s learn how to apply text formatting in PDFs using a Python PDF library. Please follow the steps given below.
- First, create a new PDF document using Document class.
- Second, pick the page where you want to place the text.
- Then, create the objects of TextFragment and set their text and other formatting options such as position, font, color, size, etc.
- After that, add the text fragments to the page using Page.paragraphs.add() method.
- Finally, call the Document.Save() method to create the PDF document.
The following code snippet shows how to apply text formatting in PDFs programmatically using Python.
You will see the output PDF as follows.
Python: Insert Images in a PDF File
We have learned to change text formatting in the previous section. Therefore, in this section, we will explain how to add images to PDF documents. Follow the steps below to do the job.
- Firstly, create a new PDF document using Document class.
- Secondly, obtain the desired page where you want to insert the image.
- Thirdly, add the image to the page using Page.add_image(file_path, rectangle), whereas Rectangle class is used to place the image on the page.
- Finally, generate the PDF document using Document.Save() method.
The following code sample shows how to add images in PDF using Python.
You will see the output PDF as follows.
Add Tables in a PDF using Python
After inserting an image, let’s add a table to your PDF file. Please write code using the steps given below:
- Create an object of Document class to create a new PDF.
- Get the page on which you want to create the table.
- Create an instance of Table class.
- Specify the borders of the table and cells using the BorderInfo class.
- Create and add a new Row to the Table.Rows collection.
- Add cells to the Row.Cells collection.
- Add the table to the page using Page.paragraphs.add() method.
- Save the PDF document using Document.Save() method.
The following code sample shows how to add a table in a PDF file using Python.
You will see the output PDF as follows.
Get a Free License
You can get a free temporary license to try the API without evaluation limitations.
Summing Up
In this article, we have explained the procedure of creating a PDF file in Python. Likewise, we have described how to add images, tables, and text formatting in a PDF document.
You may visit the documentation to learn more about the Python PDF creator library.
Please stay tuned at conholdate.com for regular updates.
Ask a Question
You can let us know your questions or queries on our forum.