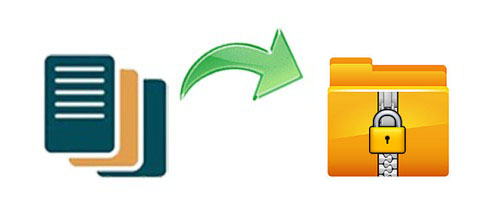
ZIP files are the most common types of archive files that are used to keep compressed files and folders into a single container. As a C# developer, you can easily create encrypted or password-protected ZIP archives programmatically using C# in your .NET applications. In this article, you will learn how to create encrypted ZIP files using C#.
The following topics are discussed/covered in this article:
- C# API to Create Encrypted ZIP Files
- Create Password-Protected ZIP Files
- Create Encrypted ZIP Files with AES Encryption
- Encrypt Folders in ZIP Files
- Encrypt Specific Files in ZIP Archives
- Create Encrypted ZIP Files with Mixed Encryption
C# API to Create Encrypted ZIP Files
For creating encrypted ZIP archives, I will be using Aspose.ZIP for .NET API. It enables you to compress files and folders and add them to ZIP archives. It also allows you to decompress archives of supported types such as ZIP, TAR, GZIP, BZ2, 7Zip, RAR, etc. The API provides protection via user-defined passwords and traditional encryption techniques using AES encryption such as AES128, AES192, and AES256.
You can either download the DLL of the API or install it using NuGet.
Install-Package Aspose.ZIP
Create Password-Protected ZIP Files
You can easily create password-protected ZIP archives programmatically by following the steps given below:
- Create an instance of the Archive class with the ArchiveEntrySettings object.
- Set password using the TraditionalEncryptionSettings object.
- Call the CreatEntry() method with the input file path to add to the archive.
- Repeat the above step to add multiple files.
- Call the Save() method with the output file path to save the output file.
The following code sample shows how to create a password-protected ZIP file using C#.
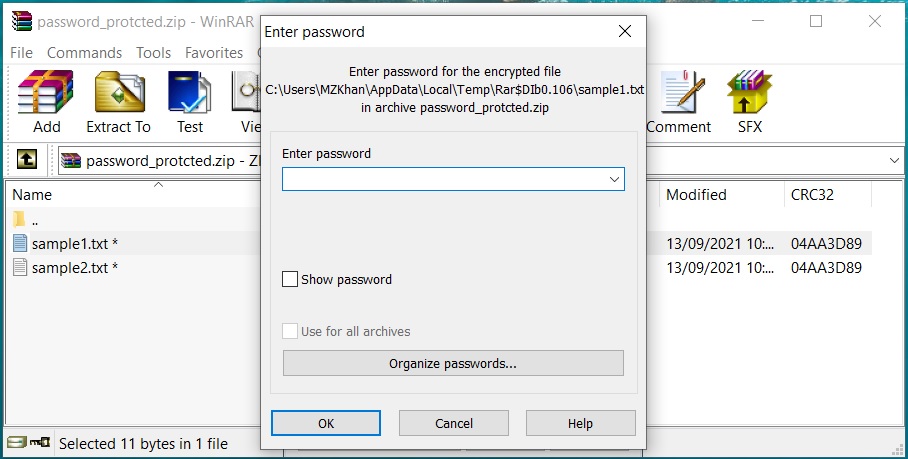
Create Password-Protected ZIP Archives
The Archive class represents a ZIP archive file. It provides several methods to create, compose, extract, or update ZIP archives. The CreatEntry() method of this class creates a single entry of a file within the archive. It takes the name of the file and the fully qualified file path as input parameters. This class also provides overloaded CreatEntry() methods to add files from stream or FileInfo. The Save() method of this class saves the ZIP archive at the specified file path.
The ArchiveEntrySettings class provides settings to compress or decompress the entries. The TraditionalEncryptionSetings class provides settings for the traditional ZipCrypto algorithm. It is a ZIP password-protection algorithm. The Password property of this class allows getting or setting a password for encryption or decryption of files and folders in a ZIP archive.
Create Encrypted ZIP Files with AES Encryption
You can encrypt your ZIP archives with AES encryption programmatically by following the steps given below:
- Create an instance of the Archive class with the ArchiveEntrySettings object.
- Set password using the AesEcryptionSettings class. Pass the password string and the EncryptionMethod as arguments to the constructor.
- Call the CreatEntry() method with the input file path to add to the archive.
- Repeat the above step to add multiple files.
- Call the Save() method with the output file path to save the output file.
The following code sample shows how to create a ZIP file encrypted with AES encryption using C#.
The AesEncryptionSettings class provides settings for AES encryption or decryption algorithm. The Advanced Encryption Standard (AES) is a symmetric encryption or decryption block cipher algorithm.
You can use the following types of encryption methods:
- Traditional — Traditional PKWARE encryption
- AES128 — Advanced Encryption Standard with key length 128 bits
- AES192 — Advanced Encryption Standard with key length 192 bits
- AES256 — Advanced Encryption Standard with key length 256 bits
Encrypt Folders in ZIP Files
You can add password-protected folders to ZIP files programmatically by following the steps given below:
- Create an instance of the Archive class with the ArchiveEntrySettings object.
- Set password using the TraditionalEncryptionSettings object.
- Call the CreatEntries() method with the folder path to add to the archive.
- Repeat the above step to add multiple folders.
- Call the Save() method with the output file path to save the output file.
The following code sample shows how to add an encrypted folder to the ZIP file using C#.
Encrypt Specific Files in ZIP Archives
You can encrypt specific files in the ZIP archives programmatically by following the steps given below:
- Create an instance of the Archive class.
- Call the CreatEntry() method with the input file path to add to the archive.
- Set password for the file using the ArchiveEntrySettings with the **TraditionalEncryptionSettings**.
- Call the CreatEntry() method with the input file path to add another file to the archive.
- Repeat above steps to add more files.
- Call the Save() method with the output file path to save the output file.
The following code sample shows how to encrypt specific files in the ZIP archive using C#.

Encrypt Specific Files in ZIP archives
Create Encrypted ZIP Files with Mixed Encryption
You can create ZIP archives containing files and folders protected with mixed encryption techniques varying for each file and folder programmatically by following the steps given below:
- Create an instance of the Archive class.
- Call the CreatEntry() method with the input file path to add to the archive.
- Set password for the file using the ArchiveEntrySettings with the AesEcryptionSettings.
- Call the CreatEntry() method with the input file path to add another file to the archive.
- Set password for the file using the ArchiveEntrySettings with the TraditionalEncryptionSettings.
- Call the CreatEntry() method with the input file path to add another file to the archive.
- Call the CreateEntries() method with the folder path to add to the archive.
- Save the output file using the Save() method with the output file path.
The following code sample shows how to create a ZIP file with mixed encryption techniques using C#.
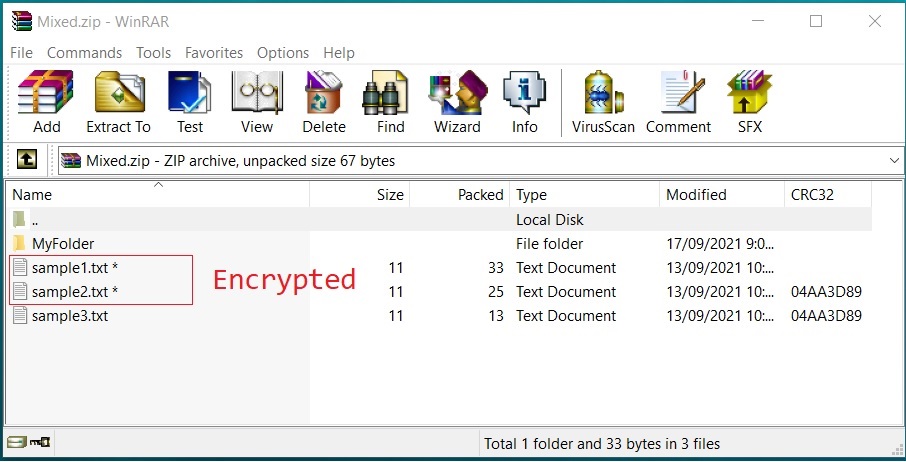
Create Encrypted ZIP File with Mixed Encryption
Get a Free License
You can try the API without evaluation limitations by requesting a free temporary license.
Conclusion
In this article, you have learned how to create encrypted ZIP files using C#. You have also learned how to create a password-protected ZIP file programmatically. Moreover, you have learned how to encrypt specific files in the ZIP archives. Furthermore, you have learned how to add password-protected folders to ZIP files. This article also explained how to create a ZIP file encrypted with mixed encryption techniques using C#. You can learn more about Aspose.ZIP for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.