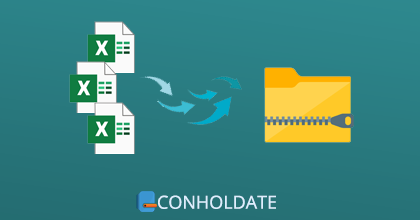
How to Compress Excel Files to ZIP in C#
The ZIP archives can contain multiple files, commonly used to reduce the size of files. At the same time, each file compresses individually, which helps to extract them and add new ones without compressing or decompressing the entire Zip archive. Nowadays, the use of Excel (XLSX or XLS) spreadsheets has increased in daily business activities. Sometimes, Excel files can get quite large, but we can reduce their size by compressing them into a ZIP archive. In this article, you will learn how to compress Excel files to a ZIP archive in C# without writing complex code. Apart from it, you will see how to get the report of compression progress while zipping the large excel files.
The following points will be covered in this article:
- C# .NET API to compress Excel files to a ZIP archive
- Add XLS or XLSX files to ZIP in C#
- Get the report of compression progress in C#
C# .NET API to compress Excel files to a ZIP archive
To compress the Excel files into a ZIP archive, we will use Aspose.ZIP for .NET. The API consists of numerous archiving features. Therefore, you can download the DLL or install it using NuGet.
Install-Package Aspose.ZIP
Add XLS or XLSX files to ZIP in C#
Suppose you have multiple Excel (XLSX or XLS) files for compressing into a ZIP archive. You can choose one of the following ways to do the job.
C# compress Excel files to ZIP using FileStream
In this way, we will use the FileStream class to add files to the ZIP archive by calling Archive.CreateEntry(String, FileStream) function.
The following are the steps to compress Excel spreadsheets to ZIP using FileStream:
- First, create an object of FileStream class for the output ZIP archive.
- Secondly, create objects of FileStream for the Excel files to be compressed.
- Then, initialize Archive class and pass it an instance of ArchiveEntrySettings class.
- After that, add FileStream objects created in step 2 using Archive.CreateEntry method.
- Finally, call Archive.Save method and use the objects of FileStream (created in step 1) and instance of ArchiveSaveOptions as parameters.
The following code shows how to compress Excel files to ZIP using FileStream.
C# compress XLSX or XLS files into ZIP using FileInfo
Alternatively, we can use the FileInfo class to add files to the ZIP archive. In this case, the files will be loaded using the FileInfo class and added to the ZIP archive by calling Archive.CreateEntry(String, FileInfo) method.
Please follow the steps mentioned below:
- Create an object of FileStream class for the output ZIP archive.
- Create objects of FileInfo for the Excel files to be compressed.
- Initialize Archive class and pass it an instance of ArchiveEntrySettings class.
- Add FileStream objects created in step 2 using Archive.CreateEntry method.
- To compress the files, call Archive.Save method and use the objects of FileStream (created in step 1) and instance of ArchiveSaveOptions as parameters.
The following code shows how to Compress XLSX or XLS files into ZIP using FileInfo.
Compress Excel spreadsheets to ZIP using the file path
The most simple way is to add an Excel file path directly to Archive.CreateEntry method:
The following are the steps to compress Excel spreadsheets to ZIP using a file path:
- Initialize Archive class.
- Add excel files using Archive.CreateEntry method.
- To compress the data, call Archive.Save method and use the name of the output file as a parameter.
The following code demonstrates how to Compress Excel spreadsheet files to ZIP using a file path.
Get the report of compression progress in C#
In the case of large Excel files, the compression process may take a long time to complete. To deal with it, the C# ZIP compression library provides [CompressionProgressed] event to post updates continuously.
The following are the steps to get the report of compression progress in C#:
- Create an object of FileStream for the large Excel file.
- Initialize Archive class and pass it an instance of ArchiveEntrySettings class.
- Obtain an object of ArchiveEntry from Archive.CreateEntry method call.
- Attach [CompressionProgressed] to the ArchiveEntry object.
- Call Archive.Save and use the name of the output file as a parameter.
The following code sample shows how to get the report of compression progress in C#:
Get a Free License
You can get a free temporary license to test the API without evaluation limitations.
Conclusion
In this article, you have learned how to compress Excel files to a ZIP archive in C# using a C# ZIP compression library. Aside from it, you got sufficient knowledge about getting compression progress report while zipping large excel files. You may visit the documentation to explore other features of C# ZIP compression API.
Ask a question
You can let us know your questions or queries on our forum.